Python - Google map API
This is just to check if my google map API is properly working before I use the API in my application. I would recommend you to do simple functionality test like this before you use it in more complicated program. Otherwise it would be difficult to troubleshoot your application problem. You would find it difficult to figure out the problem of your application comes from API operation or any other part of the program.
I assume that you know how to subscribe Google cloud service and assume that you already got subscription to it. I would not explain about how to subscribe Google Cloud service. If you are not familiar with it, try google it or check out YouTube.
How to get my API Key ?
If you go to Google Cloud home, you would get the a bunch of memu as shown below. (The exact layout may vary depending on when you go to the page, but this can be good enough for a hint.)
Select (Click) any of Google Map related menu.
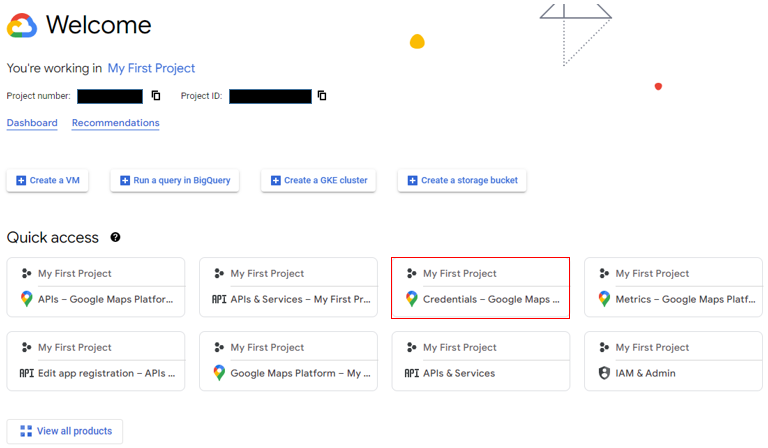
Then you would get to the page as shown below. Select (Click) on [Credentials] on the menu panel and click on [SHOW KEY], then it will show your API key.
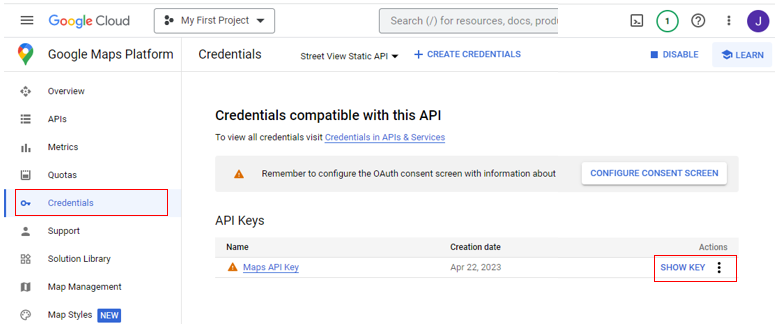
Then Copy the key and paste it into your code that requires API key.
Check it Out !!!
Before you try out the examples shown here, you need to install a few packages as below.
- googlemaps: pip install googlemaps
- folium: pip install folium
- requests: pip install requests
- Pillow (required for from PIL import Image): pip install Pillow
- matplotlib: pip install matplotlib
Followings are some of the basic test code for the API.
NOTE : Just copying this code and run it as it is would not work. You need to replace 'YOUR_API_KEY' with API key that you copied as explained above.
This code is an example of how to use the Google Maps API in Python using the googlemaps library. This is what the example code does
- The code first imports the googlemaps library, which provides a Python client for the Google Maps API.
- Then, the code creates a Client object by passing in an API key. The API key is a unique identifier that authenticates your requests to the Google Maps API and is required to use the API.
- Next, the code uses the geocode method of the gmaps object to geocode an address. Geocoding is the process of converting a street address or place name into geographic coordinates (latitude and longitude). In this case, the address is '1600 Amphitheatre Parkway, Mountain View, CA'.
- The geocode method returns a list of geocoding results. In this case, we only need the first result, so we extract it from the list using indexing.
- We then extract the latitude and longitude from the result using dictionary indexing. The latitude and longitude are stored in the lat and lng variables, respectively.
- Finally, the code prints the latitude and longitude using the print function.
Overall, this code demonstrates how to use the Google Maps API to geocode an address and extract the latitude and longitude coordinates using Python.
GooglemapTest_01.py
|
import googlemaps
# Replace "YOUR_API_KEY" with your actual API key
gmaps = googlemaps.Client(key=YOUR_API_KEY)
# Geocode an address
address = '1600 Amphitheatre Parkway, Mountain View, CA'
geocode_result = gmaps.geocode(address)
# Get the latitude and longitude of the first result
location = geocode_result[0]['geometry']['location']
lat = location['lat']
lng = location['lng']
# Print the latitude and longitude
print('The latitude and longitude of {} are:'.format(address))
print('Latitude: {}, Longitude: {}'.format(lat, lng))
|
Result |
The latitude and longitude of 1600 Amphitheatre Parkway, Mountain View, CA are:
Latitude: 37.4223878, Longitude: -122.0841877
|
Following example is similar to previous example. Actually same until it gets lattitue and longitude. The difference is that this code show the location on the map instead of the printing it out. Following is what this code is doing :
- Do the same thing as before, up until lat, lng
- The code then creates a Map object using the folium library, centered on the location of the address. The zoom_start parameter sets the initial zoom level of the map.
- Next, the code adds a marker for the location using the Marker method of the folium library. The location parameter specifies the latitude and longitude of the marker, and the popup parameter sets the text that appears when the marker is clicked.
- Finally, the code saves the map as an HTML file using the save method of the Map object.
GooglemapTest_02.py
|
import googlemaps
import folium
# Replace "YOUR_API_KEY" with your actual API key
gmaps = googlemaps.Client(key=YOUR_API_KEY)
# Geocode an address
address = '1600 Amphitheatre Parkway, Mountain View, CA'
geocode_result = gmaps.geocode(address)
# Get the latitude and longitude of the first result
location = geocode_result[0]['geometry']['location']
lat = location['lat']
lng = location['lng']
# Create a map centered on the location
map = folium.Map(location=[lat, lng], zoom_start=15)
# Add a marker for the location
folium.Marker(location=[lat, lng], popup=address).add_to(map)
# Save the map as an HTML file
map.save('map.html')
|
Result |
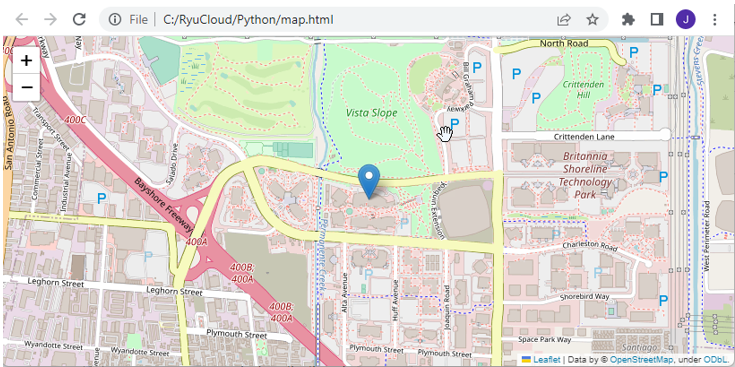
|
The goal of the following example is same as previous one. That is, to mark the location of a certain address on the map. The difference is to display the map directly onto Pythong figure window instead of generating a separte html file and open the html in a browser.
Following is overall procedure of the code.
- First, the code imports the required libraries requests, Pillow, and matplotlib.pyplot.
- The code then sets the Google Maps API key to a variable named api_key. This API key is required to use the Google Maps API.
- Next, the code specifies an address to display on the map by setting the address variable to 'Tischart Cres Kanata, ON, Canada'.
- The code constructs a URL for the static map image using the Google Maps Static Maps API. The URL includes the address to display, the zoom level, the size of the image, and the map type. A red marker is added to the map at the location of the address.
- The code then sends a GET request to the API using the requests library and stores the response in a variable named response. The response is then converted to an image using the Image.open method of the Pillow library.
- Finally, the image is displayed in a Python plot using the imshow method of the matplotlib.pyplot library. The axis method is used to turn off the display of axes on the plot. The show method is called to display the plot.
GooglemapTest_03.py
|
import requests
from PIL import Image
from io import BytesIO
import matplotlib.pyplot as plt
# Replace "YOUR_API_KEY" with your actual API key
api_key = 'YOUR_API_KEY
# Geocode an address
address = 'Tischart Cres Kanata, ON, Canada'
# Construct the URL for the static map image
url = 'https://maps.googleapis.com/maps/api/staticmap?center={}&zoom=15&size=640x640&maptype=roadmap&markers=color:red|{}'.format(address, address)
url += '&key=' + api_key
# Get the static map image
response = requests.get(url)
img = Image.open(BytesIO(response.content))
# Display the image in a Python plot
plt.imshow(img)
plt.axis('off')
plt.show()
|
Result |
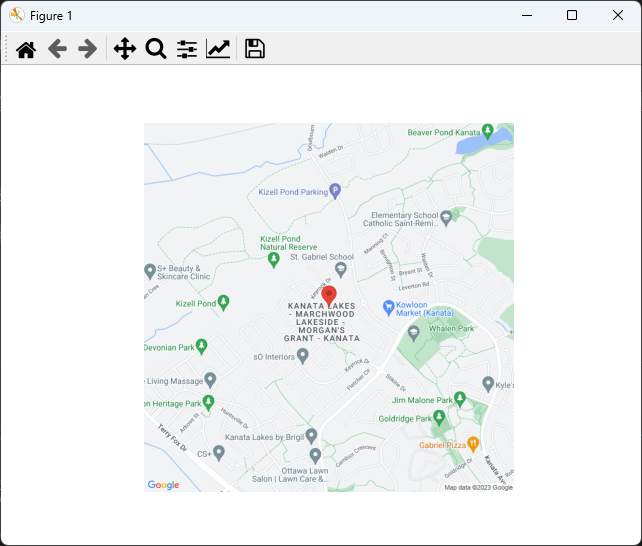
|
|
|